Utility
Description
The artifact plantuml-generator-util contains two Utility classes:
-
PlantUMLClassDiagramGenerator
for generation of class diagrams -
PlantUMLSequenceDiagramGenerator
for generation of sequence diagrams
Both can be used to generate a PlantUML diagram text out of existing java classes. You can use this string as input for the PlantUML tools or as part of your "living" documentation (for example with asciidoc)
Usage
To use this utility library you need to add the plantuml-generator-util.jar to your classpath.
If you use maven as build tool this is easy, just add the following dependency:
<dependency>
<groupId>de.elnarion.util</groupId>
<artifactId>plantuml-generator-util</artifactId>
<version>{generatorversion}</version>
</dependency>
to your pom.xml .
Afterwards just create a PlantUMLClassDiagramConfigBuilder for class diagrams or a PlantUML SequenceDiagramConfigBuilder for sequence diagrams. Add them to the corresponding generator class and start the generation of the diagram text:
Class diagram
List<String> scanPackages = new ArrayList<>();
scanPackages.add("de.elnarion.test.domain.t0001");
List<String> hideClasses = new ArrayList<>(); (1)
hideClasses.add("de.elnarion.test.domain.ChildB");
PlantUMLClassDiagramConfigBuilder configBuilder = new PlantUMLClassDiagramConfigBuilder(scanPackages) (2)
.withHideClasses(hideClasses); (3)
PlantUMLClassDiagramGenerator generator = new PlantUMLClassDiagramGenerator(configBuilder.build()); (4)
String result = generator.generateDiagramText(); (5)
String expectedDiagramText = IOUtils.toString(Objects.requireNonNull(classLoader.getResource("class/0001_general_diagram.txt")),
StandardCharsets.UTF_8);
assertNotNull(result);
assertNotNull(expectedDiagramText);
assertEquals(expectedDiagramText.replaceAll("\\s+", ""), result.replaceAll("\\s+", ""));
the result of this generation is
@startuml
class de.elnarion.test.domain.t0001.BaseAbstractClass {
{method} +doSomething () : void
{method} +doSomethingElse () : void
{method} +doSomethingWithParameter ( paramString1 : String ) : void
{method} +doSomethingWithReturnValue () : String
}
interface de.elnarion.test.domain.t0001.BaseInterface {
{method} {abstract} +doSomething () : void
{method} {abstract} +doSomethingWithParameter ( paramString1 : String ) : void
{method} {abstract} +doSomethingWithReturnValue () : String
}
class de.elnarion.test.domain.t0001.ChildA {
}
class de.elnarion.test.domain.t0001.ChildB {
}
class de.elnarion.test.domain.t0001.Util {
}
de.elnarion.test.domain.t0001.BaseAbstractClass ..|> de.elnarion.test.domain.t0001.BaseInterface
de.elnarion.test.domain.t0001.ChildA --|> de.elnarion.test.domain.t0001.BaseAbstractClass
de.elnarion.test.domain.t0001.ChildB --> de.elnarion.test.domain.t0001.Util : util
de.elnarion.test.domain.t0001.ChildB --|> de.elnarion.test.domain.t0001.BaseAbstractClass
hide de.elnarion.test.domain.ChildB
@enduml
which is rendered in PlantUML this way:
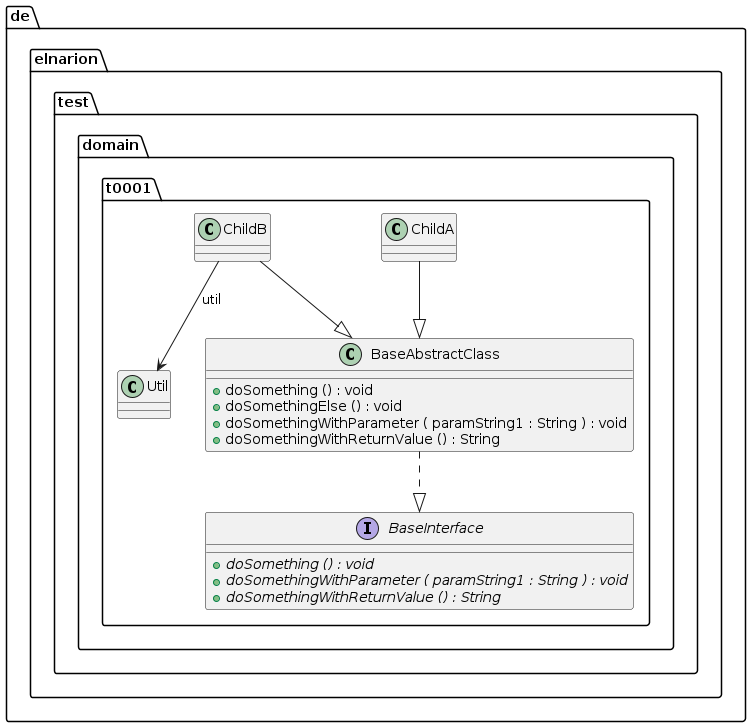
Sequence diagram
// ARRANGE
PlantUMLSequenceDiagramConfigBuilder builder = new PlantUMLSequenceDiagramConfigBuilder(CallerA.class.getName(), (1)
"callSomething"); (2)
PlantUMLSequenceDiagramGenerator generator = new PlantUMLSequenceDiagramGenerator(builder.build()); (3)
String expectedDiagramText = IOUtils.toString(Objects.requireNonNull(classLoader.getResource("sequence/0001_basic_caller_test.txt")),
StandardCharsets.UTF_8);
// ACT
String generatedDiagram = generator.generateDiagramText(); (4)
// ASSERT
assertAll(() -> assertNotNull(generatedDiagram), () -> assertEquals(expectedDiagramText.replaceAll("\\s+", ""),
generatedDiagram.replaceAll("\\s+", "")));
the result of this generation is
@startuml
participant CallerA
participant CallerB
activate CallerA
CallerA -> CallerB : callSomething
activate CallerB
CallerB -> CallerB : privateMethodCall
activate CallerB
CallerB --> CallerB
deactivate CallerB
CallerB -> CallerB : protectedMethodCall
activate CallerB
CallerB --> CallerB
deactivate CallerB
CallerB --> CallerA
deactivate CallerB
CallerA -> CallerB : callSomethingElse
activate CallerB
CallerB --> CallerA
deactivate CallerB
deactivate CallerA
@enduml
which is rendered in PlantUML this way:
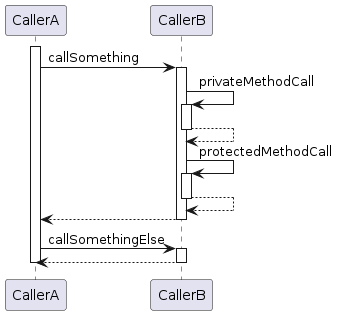
Upgrade from 1.x to 2.x
With the new version 2.x some classes moved to other packages to better distinguish between classes for class diagrams and those for sequence diagrams.
-
the following class moved to de.elnarion.util.plantuml.generator.classdiagram
-
PlantUMLClassDiagramGenerator
-
-
the following classes moved to de.elnarion.util.plantuml.generator.classdiagram.config
-
PlantUMLClassDiagramConfigBuilder
-
ClassifierType
-
VisibilityType
-
So you need to switch to the new packages.
Furthermore the deprecated constructors of PlantUMLClassDiagramGenerator have been removed. Use the PlantUMLClassDiagramConfigBuilder instead.
Upgrade from 2.x to 3.x
With the new version 3.x the configuration parameter addJavaxValidationAnnotations of the PlantUMLClassDiagramGenerator has changed to addValidationAnnotations and now supports javax and jakarta namespace for validations. So you need to change your configuration if you used this parameter.